1. import libraries
import math
math.sqrt(25)
# import a function from math from math import sqrt sqrt(25)
# import multiple functions at once from math from math import cos, floor
import os
os.getcwd() # in R, getwd()
os.chdir(“c:/data/pydata”) # in R, setwd(“c:/data/rdata”)
import pandas as pd
bmi = pd.read_csv(“bmi.csv”)
2. comment
# comments in line 파이썬 주석은 아래처럼 달 수 있다.
“””
Comments in sentences
Brian Heinold, A Practical Introduction to Python Programming, 2012.
Edouard Duchesnay, Tommy Löfstedt. Statistics and Machine Learning in Python, 2018.
“””
help(“modules”) : 설치된 모듈 목록 보기
3. pip
Python 패키지를 설치하고 관리하는 프로그램이다
# 파이썬으로 pip install 하고 업데이트 하기
python –m pip install –upgrade pip
pip install mglearn
pip install graphviz
4. 기본 연산
- **라는 연산자가 있다. 이 연산자는 x**y처럼 사용했을 때 x의 y제곱(xy) 값을 돌려준다.
- %는 나눗셈의 나머지 값을 돌려주는 연산자이다. 7을 3으로 나누면 나머지는 1이 될 것이고 3을 7로 나누면 나머지는 3이 될 것이다.
- / 연산자를 사용하여 7 나누기 4를 하면 그 결과는 예상대로 1.75가 된다.
# Number
10**3
10 / 4
10 / float(4)
5 % 4
10 // 4
# Boolean operations
# comparisons (these return True)
5>3
5 >= 3
5 != 3
5 == 5
# boolean operations (these return True)
5 > 3 and 6 > 3 5 > 3 or 5 < 3 not
False False or not False and True
5. data types
자료형이란 프로그래밍을 할 때 쓰이는 숫자, 문자열 등 자료 형태로 사용하는 모든 것을 뜻한다.
- 숫자형(Number)이란 숫자 형태로 이루어진 자료형
- 정수형(Integer)이란 말 그대로 정수를 뜻하는 자료형을 말한다.
- 실수형(Floating-point)은 소수점이 포함된 숫자를 말한다.
- 문자열(String)이란 문자, 단어 등으로 구성된 문자들의 집합을 의미한다.
# determine the type of an object
type(2) type(2.0) type('two') type(True) type(None)
# returns 'int'
# returns 'float'
# returns 'str'
# returns 'bool'
# returns 'NoneType'
# check if an object is of a given type
isinstance(2.0, int) # returns False isinstance(2.0, (int, float)) # returns True
# convert an object to a given type
float(2)
int(2.9)
str(2.9)
# zero, None, and empty containers are converted to False bool(0)
bool(None)
bool('') bool([]) bool({})
# empty string
# empty list
# empty dictionary
파이썬에서 문자열을 만드는 방법은 총 4가지이다.
1) 큰따옴표(")로 양쪽 둘러싸기
"Hello World"
2) 작은따옴표(')로 양쪽 둘러싸기
'Python is fun'
3) 큰따옴표 3개를 연속(""")으로 써서 양쪽 둘러싸기
"""Life is too short, You need python"""
4) 작은따옴표 3개를 연속(''')으로 써서 양쪽 둘러싸기
'''Life is too short, You need python'''
6. 변수
파이썬은 자바와 다르게 변수를 리스트[], 튜플(), 딕셔너리{}에 담는다.
- list : 대괄호 []로 묶어서 생성한다.
append()로 object를 추가, remove()로 제거할 수 있다.
# empty list
empty = []
# empty = list()
empty.append(23)
empty.append(45) empty
Out[20]: [23, 45]
# list slicing [start:end:stride]
weekdays = ['mon','tues','wed','thurs','fri']
weekdays[0] # element 0
weekdays[0:3] # elements 0, 1, 2
weekdays[:3] # elements 0, 1, 2
weekdays[3:] # elements 3, 4
weekdays[-1] # last element (element 4)
weekdays[::2] # every 2nd element (0, 2, 4)
weekdays[::-1] # backwards (4, 3, 2, 1, 0)
# sort a list
simpsons.sort()
simpsons.sort(reverse=True) # sort in reverse
simpsons.sort(key=len) # sort by a key
# conatenate +, replicate *
[1, 2, 3] + [4, 5, 6]
["a"] * 2 + ["b"] * 3
- tuple : 괄호()로 묵어서 생성한다.
elements 추가 및 삭제가 불가능하다, del()로 튜플 전체를 삭제해야 한다.
# Tuples are enclosed in paranthesis
mytuple=(1,3,7,6,"test") print(mytuple)
# Lists are enclosed in square bracket
mylist = [1, 2, 7, 4, 12 ]
#Dictionary - These are similar to name-value pairs
mydict={'Name':'Ganesh','Age':54,'Occupation':'Engineer'}
print(mydict)
print(mydict['Age'])
# No of elements in tuples, lists and dictionaries can be got with len()
print("Length of tuple=",len(mytuple))
print("Length of list =", len(mylist))
print("Length of dictionary =",len(mydict))
# create a tuple
digits = (0, 1, 'two') # create a tuple directly
digits = tuple([0, 1, 'two']) # create a tuple from a list
# examine a tuple
digits[2] # returns 'two'
len(digits) # returns 3
digits.count(0) # counts the number of instances of that value (1)
digits.index(1) # returns the index of the first instance of that value (1)
- dictionary : 중괄호{}로 묶어서 생성한다.
(key : value) 쌍으로 구성되어있으며 key는 고유값이다.
len()으로 길이를 확인할 수 있다.
# create a dictionary (two ways)
family = {'dad':'homer', 'mom':'marge', 'size':6}
family = dict(dad='homer', mom='marge', size=6)
# examine a dictionary
family['dad'] # returns 'homer'
len(family) # returns 3
family.keys() # returns list: ['dad', 'mom', 'size']
family.values() # returns list: ['homer', 'marge', 6]
family.items() # returns list of tuples:
# [('dad', 'homer'), ('mom', 'marge'), ('size', 6)]
'mom' in family # returns True
'marge' in family # returns False (only checks keys)
- set : 중괄호 {}로 묶어서 생성한다.
list와 달리 중복과 순서가 없는 것이 특징이다.
# create a set
languages = {'python', 'r', 'java'} # create a set directly
snakes = set(['cobra', 'viper', 'python']) # create a set from a list
# examine a set
len(languages) # returns 3
'python' in languages # returns True
# set operations 1
languages & snakes # returns intersection: {'python'}
languages | snakes # returns union: {'cobra', 'r', 'java', 'viper', 'python'}
languages - snakes # returns set difference: {'r', 'java'}
snakes - languages # returns set difference: {'cobra', 'viper'}
# set operations 2
s1 = {1,2,3,4,5}
s2 = {2,4,6}
print(s1.intersection(s2)) # 교집합 {2, 4}
print(s1.union(s2)) # 합집합
{1, 2, 3, 4, 5, 6}
7. if(조건문)
조건문은 기본적으로 if esle 구조를 가지고있다.
파이썬에서는 키 4개를 이용해 반드시 들여쓰기 한 후에 print("코멘트")이라고 작성해야 한다.
x=3
# if statement
if x > 0:
print('positive')
# if/else statement
if x > 0:
print('positive')
else:
print('zero or negative')
# if/elif/else statement
if x > 0:
print('positive')
elif x == 0:
print('zero) else:
print('negative')
8. Loops (반복문)
반목문은 기본적으로 for문과 while문을 사용한다.
- for문을 사용하면 실행해야 할 문장을 여러 번 반복해서 실행시킬 수 있다.
# range returns a list of integers
range(0, 3) # returns [0, 1, 2]: includes first value but excludes second value
range(0, 5, 2) # returns [0, 2, 4]: third argument specifies the 'stride'
# 아래 예는 대괄호([ ])사이에 있는 값들을 하나씩 출력한다.
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit.upper())
for fruit in fruits:
if fruit == 'banana':
print("Found the banana!")
break # exit the loop and skip the 'else' block # 분기문
else: # break 가 아닌 경우에만 else 블록 실행한다.
# this block executes ONLY if the for loop completes without hitting 'break'
print("Can't find the banana")
count = 0
while count < 5:
print("This will print 5 times")
count += 1 # equivalent to 'count = count + 1' #count 값이 1씩 증가한다.
- Lambda
Lambda operations all you to create small anonymous function which computes something
# operations on list
a = [5,2,3,1,7]
b = [1,5,4,6,8]
# Create a lambda function to add 2 numbers
add_fct = lambda x,y:x+y
add_fct(a,b)
Out[12]: [5, 2, 3, 1, 7, 1, 5, 4, 6, 8]
# Add all elements of lists a and b
print(list(map(add_fct, a,b)))
[6, 7, 7, 7, 15]
- Ref: Practical machine learning with R and Python:3rd ed., Tinniam V Ganesh
9. Functions(함수)
파이썬에서 def는 함수를 만들 때 사용하는 예약어이다.
예약어란 프로그래밍 언어에서 이미 문법적인 용도로 사용하고 있는 단어를 말한다.
default arguments
def power_this(x, power=2):
return x ** power
power_this(2) # 4
power_this(2, 3) # 8
# return two values from a single function
def min_max(nums):
return min(nums), max(nums)
# return values can be assigned to a single variable as a tuple
nums = [1, 2, 3]
min_max_num = min_max(nums) # min_max_num = (1, 3)
10. Object oriented programming(OOP)
class 객체지향프로그래밍
import math
# Inheritance + Encapsulation
class Square():
def __init__(self, width):
self.width = width
def area(self):
return self.width ** 2
class Disk():
def __init__(self, radius):
self.radius = radius
def area(self):
return math.pi * self.radius ** 2
shapes = [Square(2), Disk(3)]
# Polymorphism
print([s.area() for s in shapes])
수행결과
[4, 28.274333882308138]
11. Read text(excel) files with pandas
import os
import pandas as pd
import matplotlib.pyplot as plt
# Set the current working directory
os.chdir(“c:/data/pydata”)
os.getcwd() # 'c:\\data\\pydata' bmi.csv
# data = pd_read.csv(‘c:/data/pydata/bmi.csv’)
data = pd.read_csv(“bmi.csv”)
data.head()
Out[13]:
height weight
0 181 78
1 161 49
2 170 52
3 160 53
4 158 50
weig = data[‘weight’]
heig = data[‘height’]
bmi = weig/(heig/100)**2
plt.scatter(heig, weig)
plt.show()
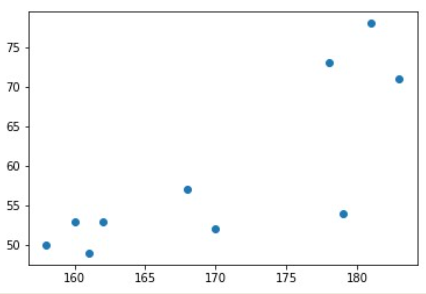
import os
import pandas as pd
import matplotlib.pyplot as plt
# Set the current working directory
os.chdir(“c:/data/pydata”)
os.getcwd() # 'c:\\data\\pydata'
beer = pd.read_excel(“beer.xlsx, sheet_name=‘Beer’)
beer.head()
beer['cost']
12. Numpy
NumPy is one of the most fundamental package for scientific computing with Python. Numpy includes the support for handling large, multi- dimensional arrays and matrices, along with a large collection of high- level mathematical functions to operate on these arrays.
import numpy as np
#Create a 1d numpy array
data1 = [6, 7.5, 8, 0, 1]
arr1 = np.array(data1)
print(arr1)
# Create numpy array in a single line
import numpy as np
arr1= np.array([6, 7.5, 8, 0, 1])
#Print the array
print(arr1)
### 2D array
#Create a 2d numpy array
import numpy as np
data2 = [[1, 2, 3, 4], [5, 6, 7, 8]]
arr2 = np.array(data2)
# Print the 2d array
print(arr2)
'Programming > Python' 카테고리의 다른 글
[Python] colab에서 python으로 url 날려서 파일 다운로드 크롤링 하기 (0) | 2022.03.17 |
---|---|
[Python] 코랩과 파이썬을 이용해 구글 드라이브에 폴더 만들고 파일 쌓고 불러오기 (0) | 2022.03.17 |
[Python] 크롤링 돌리면서 생긴 에러 (0) | 2022.03.16 |
[Python] colab에서 폴더 생성하고 sqlite3로 테이블 생성하기 with logging (0) | 2022.03.15 |
[Python] 출력 (0) | 2022.03.07 |
댓글